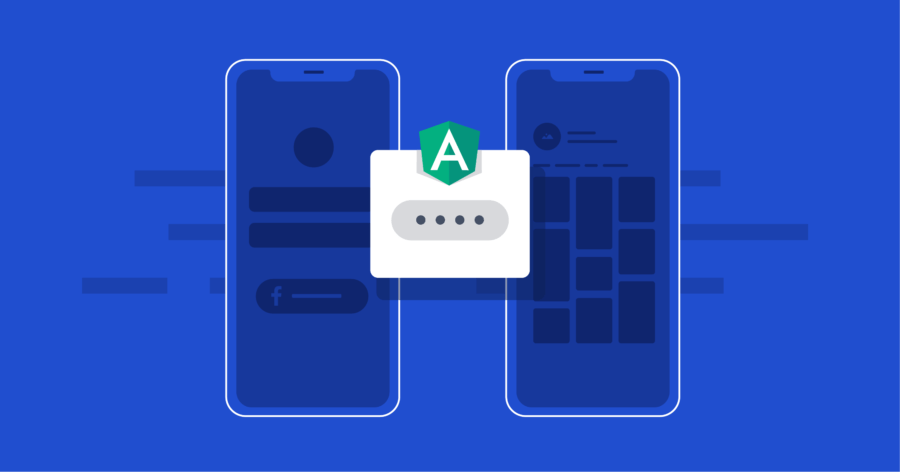
Facebook Authentication With Angular JS And Satellizer
hello there, today we are going to learn how can we do facebook authentication using angular js and Satellizer. before we begin the practical part let’s see what is Satellizer and how can we use it for Facebook authentication.
what is satelizer?
Satellizer is an end-to-end, token-based authentication module for AngularJS with built-in support for Google, Facebook, Linked In, and other social media, etc as well as Email and Password sign-in.
Satellizer is simple to use.
However, you are not limited to the sign-in options above, in fact, you can add any OAuth 1.0 or OAuth 2.0 provider bypassing provider-specific information in the app config block.
you can learn more about satelizer here.
satelizer installation and Requirements.
in this tutorial, we are gonna use Satellizer through CDN.
<script src="https://cdn.jsdelivr.net/satellizer/0.15.5/satellizer.min.js"></script>
another installation process is also there for the node JS or if you want to install it in the browser you can visit here.
also, you can check the requirements for mobile apps there.
so now we are going to do Facebook authentication with angular js and Satellizer, first of all, we are going to do the front-end part then we are going to do scripting. so let’s create an index page with a Facebook login button then we will do authentication.
Let’s create front-end For the tutorial
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script>
<script src="https://cdn.jsdelivr.net/satellizer/0.15.5/satellizer.min.js"></script>
<style>
body {
font-family: Arial, Helvetica, sans-serif;
}
* {
box-sizing: border-box;
}
/* style the container */
.container {
position: relative;
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px 0 30px 0;
width: 800px;
float: right;
height: 400px;
top: 100px;
}
/* style inputs and link buttons */
input,
.btn {
width: 100%;
padding: 12px;
border: none;
border-radius: 4px;
margin: 5px 0;
opacity: 0.85;
display: inline-block;
font-size: 17px;
line-height: 20px;
text-decoration: none; /* remove underline from anchors */
}
input:hover,
.btn:hover {
opacity: 1;
}
/* add appropriate colors to fb, twitter and google buttons */
.fb {
background-color: #3B5998;
color: white;
}
.twitter {
background-color: #55ACEE;
color: white;
}
.google {
background-color: #dd4b39;
color: white;
}
/* style the submit button */
input[type=submit] {
background-color: #04AA6D;
color: white;
cursor: pointer;
}
input[type=submit]:hover {
background-color: #45a049;
}
/* Two-column layout */
.col {
float: left;
width: 50%;
margin: auto;
padding: 0 50px;
margin-top: 6px;
}
/* Clear floats after the columns */
.row:after {
content: "";
display: table;
clear: both;
}
/* vertical line */
.vl {
position: absolute;
left: 50%;
transform: translate(-50%);
border: 2px solid #ddd;
height: 275px;
}
/* text inside the vertical line */
.vl-innertext {
position: absolute;
top: 50%;
transform: translate(-50%, -50%);
background-color: #f1f1f1;
border: 1px solid #ccc;
border-radius: 50%;
padding: 8px 10px;
}
/* hide some text on medium and large screens */
.hide-md-lg {
display: none;
}
/* bottom container */
.bottom-container {
text-align: center;
background-color: #666;
border-radius: 0px 0px 4px 4px;
}
/* Responsive layout - when the screen is less than 650px wide, make the two columns stack on top of each other instead of next to each other */
@media screen and (max-width: 650px) {
.col {
width: 100%;
margin-top: 0;
}
/* hide the vertical line */
.vl {
display: none;
}
/* show the hidden text on small screens */
.hide-md-lg {
display: block;
text-align: center;
}
}
</style>
</head>
<body ng-app="myApp" ng-controller="LoginCtrl">
<div class="container">
<form method="post">
<div class="row">
<h2 style="text-align:center">Login with Social Media or Manually</h2>
<div class="vl">
<span class="vl-innertext">or</span>
</div>
<div class="col">
<button class="fb btn" ng-click="authenticate('facebook')">
<i class="fa fa-facebook fa-fw"></i> Login with Facebook
</button>
<a href="#" class="twitter btn">
<i class="fa fa-twitter fa-fw"></i> Login with Twitter
</a>
<a href="#" class="google btn"><i class="fa fa-google fa-fw">
</i> Login with Google+
</a>
</div>
</form>
<div class="col">
<div class="hide-md-lg">
<p>Or sign in manually:</p>
</div>
<form method="post">
<input type="text" name="username" placeholder="Username" required>
<input type="password" name="password" placeholder="Password" required>
<input type="submit" value="Login">
</form>
</div>
</div>
</form>
</div>
<script type="text/javascript" src="app.js"></script>
</body>
</html>
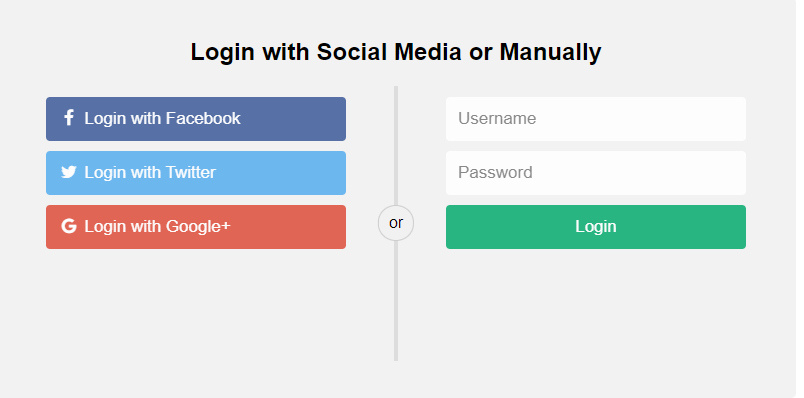
this is the front-end part of the UI. now when the user clicks sign in with Facebook then there should be a popup for authentication so now let’s create this first.
so if you want to make a Facebook authentication then you must have an account on the Facebook developer console website.
so first create an account in the Facebook developer console then create a new application fill the all the forms and required fields.
then you have to go to your application and click on the library section where you can find the Facebook login library and you have to install your app in code using application code.
Let’s create a script for authentication.
there is all the script available here. you just have to put your application code then see if it works or not but here we have created a script like this, which is only for Facebook authentication.
the script which we have written is in angular JS so must have a knowledge of angular js, so you need to learn angular js, some basic funda of angular js is must required.
var MyApp = angular.module('myApp', ['satellizer'])
MyApp.config(function($authProvider) {
var AuthUrl = 'http://localhost/facebookAuth/index.php';
$authProvider.facebook({
clientId: 'your application id',
name: 'facebook',
url: AuthUrl,
authorizationEndpoint: 'https://www.facebook.com/v2.5/dialog/oauth',
requiredUrlParams: ['display', 'scope'],
scope: ['email'],
scopeDelimiter: ',',
display: 'popup',
oauthType: '2.0',
popupOptions: { width: 580, height: 400 }
});
});
MyApp.controller('LoginCtrl', function($scope, $auth)
{
$scope.authenticate = function(provider) {
$auth.authenticate(provider)
.then(function (response){
console.log("connected successfully");
};
});
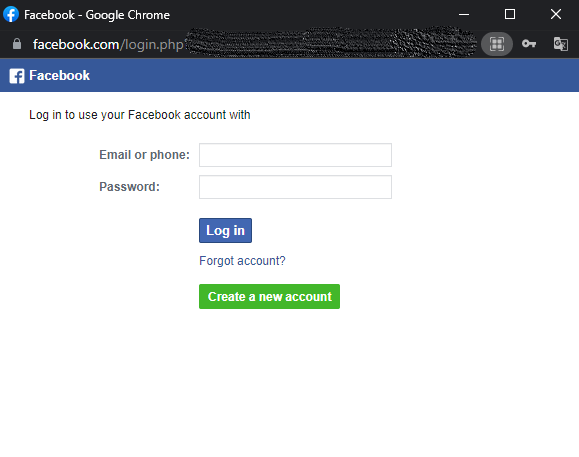
once your account is verified you can do what you want to do after authentication.
that’s it, for now, check our previous blogs here.